Arduino board
Arduino Nano v2.3 manual (pdf)
Arduino and shift register 74HC595
74HC595 Datasheet (pdf)
74HC595 Explanation
Youtube tutorial with buttons:
Tutorial with arduino - I was using that.
My version on a breadboard:
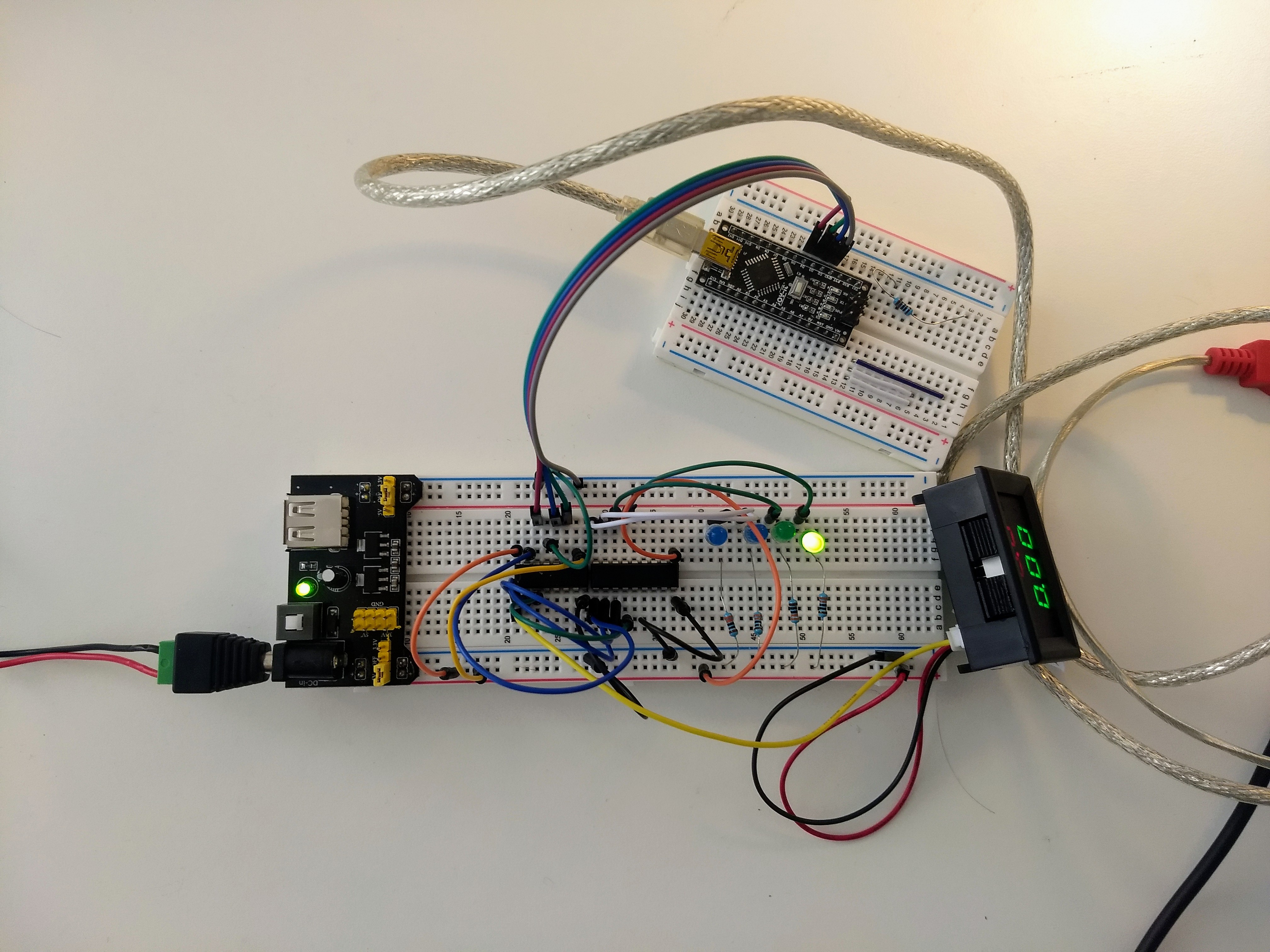
Code:
|
|
Alternatives
- More powerful shift register: TPIC6B595
- PWM: TLC5940, TLC5947
- PWM with i2c: PCA9685, PCA9635
PWM via shift register
PWM Through a 74HC595 Shift Register at forum.arduino.cc
Issues with upload to Arduino Nano v3
Trying to connect to my Arduino clone (JOY-IT Arduino-compatible Nano V3 Board with ATmega328P-AU) from Ubuntu 18 I got a strange error messages.
There are three ways you can install Arduin IDE in ubuntu 18:
sudo apt install arduino
- install version 1.0.5 as on 2019-07-04arduino-mhall119
1.8.5 via snap andUbuntu Software
app- arduino IDE 1.8.9 from https://www.arduino.cc/en/guide/linux
First of all I had to add a current user to dialout
group and logout-login – that’s fine:
|
|
Then all the tutorials require for checking
|
|
And I don’t have that. I have USB0 instead:
|
|
When I tried to upload some simple programms to the board I got: *
avrdude: ser_open(): can't open device "/dev/ttyUSB0": Permission denied
- For version from sudo apt install:
arduino nano avrdude: ser_open(): can't open device "COM1": No such file or directory
That version was way too old.
- For the version from website I got
Build options changed, rebuilding all
Sketch uses 994 bytes (3%) of program storage space. Maximum is 30720 bytes.
Global variables use 9 bytes (0%) of dynamic memory, leaving 2039 bytes for local variables. Maximum is 2048 bytes.
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 1 of 10: not in sync: resp=0x00
avrdude: stk500_recv(): programmer is not responding
avrdude: stk500_getsync() attempt 2 of 10: not in sync: resp=0x00
That was almost fine.
To fix that I had to chose Tools -> Processor -> ATmega 328P (old bootloader) instead of just “ATmega 328P”